Know Vue.js Lifecycle hooks
Lifecycle hooks are very important in any component created in Vue.js or any other programming language. the developer must know when the component is created when the component is added to the DOM, updated and destroyed. lifecycle hooks are the window process behind the scene when you created a component.
Vue Js have a very clear and easy to understand lifecycle hook the following image is from Vue js official documentation. You can go and check here
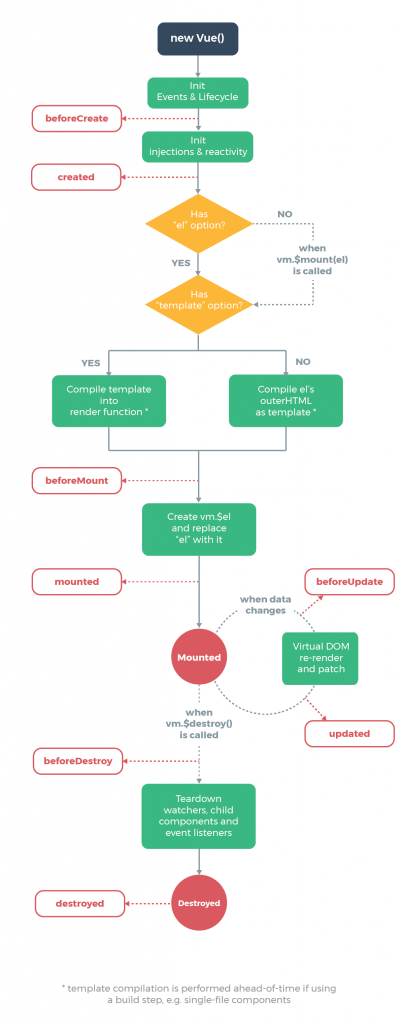
creation (To make rady)
These hooks are very first to run in your component. it allows you to perform the action before you component added to the DOM. it is quite different than other hooks it also runs during serverside rendering.
This is very handy if you need to set up things during client-side rendering and server-side rendering to your component.
Inside the creation hooks, you did not have access to the DOM or mounting element (this.$el).
1. beforeCreate
The beforeCreate Hook runs at the very first during the initializing of DOM. the events are not set up at this moment and data are not reactive.
example:
<script>
export default {
beforeCreate() {
console.log(‘i am calling from before create’)
}
}
</script>
2. created
In this hook, you can access reactive data and events but keep in mind that the virtual DOM is not yet mounted or rendered.
<script>
export default {
data() {
return {
message: ‘hello world’
}
},
computed: {
messageComputed() {
console.log('I change when this.message changes.')
return this.property
}
},
created() {
this.message = ‘i am changing from created’
console.log(‘messageComputed will update, because this.message is reactive.’)
}
}
</script>
Mounting (DOM Initialize)
This is the most used hooks among all. mounting hooks give access to the component before and after the first render. but it doesn’t run during serverside rendering.
It is good practice to use if you need to modify the DOM just before and after the rendering.
It is not a good practice to use when we need to fetch data during initialization. instead of using mounting it is better to use created.
1. beforeMount
It runs just before the initial render and after the template complied. but it cannot be called during serverside rendering.
example:
<script>
export default {
beforeMount() {
console.log(‘i am just before mounted’)
}
}
</script>
2. Mounted
This hook is called after the instance are mounted when $ek ropery is replaced y the newly created vm.$el property. the component becomes fully functional after this hook is called. this hook is mainly used to fetch data for the initialized component from the server.
Example
<script>
export default {
mounted() {
console.log(‘i am mounted hooks and called from mounted’) // I'm text inside the component.
}
}
</script>
Updating
This hook is called when there is something change on the initialized component and need to re-render the instance.
it is best to use when the component needs to re-render.
it is bad practice to use when reactive are changed on the component. it is better to use a watch or computed instead of these hooks.
1. beforeUpdate
This hook runs just after something change on rendered component and update begin. just before the DOM re-render. it gives to access to get a new state of any reactive data on the rendered component before actual render. it cannot be called during serverside rendering.
example:
<script>
export default {
data () {
return {
increment : 0
}
},
beforeUpdate (){
console.log(this.increment)
// the increment will log in every secend with added value
},
}
created() {
setInterval(()=> {
this.increment++
}, 1000)
}
</script>
2. Updated
This hook run after data changes on rendered DOM and DOM will re-renders. updated hook doesn’t guarantee that all the child has been re-rendered. actually is not best practice to use updated hooks it is better to use computed or watch instead of updated. this hook cannot be called during the serverside rendering
<template>
<p ref="update">{{increment}}</p>
</template>
<script>
export default {
data() {
return {
increment: 0
}
},
updated() {
// Fired every second, should always be true
console.log(+this.$refs['update'].textContent === this.increment)
},
created() {
setInterval(() => {
this.increment++
}, 1000)
}
}
</script>
Destroy (Trashing)
Destroy hooks allow us to trash our loaded component. it is best to use when you want to destroy all the data from local storage where you have saved.
1. beforeDestroy
This is Called just before a Vue instance is destroyed. In this stage the instance is still fully functional we can still perform other stuff within the instance.
<script>
export default {
data() {
return {
increment: 0
}
},
beforeDestroy() {
// Clean up the increment.
// (In this case, effectively nothing)
this.increment = null
delete this.increment
}
}
</script>
2. destroyed
Called after a rendered instance has been destroyed. when this hook is called all the instance related child instance event listeners and directive will be destroyed.
<script>
import DestroyEverything from ‘./everything’
export default {
destroyed() {
console.log(this) // There's practically nothing here!
DestroyEverything.informThem(‘everything destroyed')
}
}
</script>
Conclusion
This is the life cycle hooks of Vue.js Vue.js is very Progressive adoptable framework. you can find a complete documentation of following link official Vue.js documentation website
Thank you for reading my blog please comment down what do you think about this article and provide your feedback so that I can update the blog regularly